// You can also construct a button in the form, and use GetCommand to receive the contents of the cmd attribute.
var table = {
type: 'table',
title: 'position operation',
cols: ['Column1', 'Column2', 'Action'],
rows: [
['abc', 'def', {'type':'button', 'cmd': 'coverAll', 'name': 'close position'}],
]
};
LogStatus('`' + JSON.stringify(table) + '`')
// Or construct a separate button
LogStatus('`' + JSON.stringify({'type':'button', 'cmd': 'coverAll', 'name': 'close position'}) + '`')
// Button styles can be customized (bootstrap's button attributes)
LogStatus('`' + JSON.stringify({'type':'button', 'class': 'btn btn-xs btn-danger', 'cmd': 'coverAll', 'name': 'close position'}) + '`')
The API documentation shows that displaying tables, strings, images, charts, etc. in the strategy status bar is accomplished by calling the API function:
LogStatus
. We can also set up an interactive button by constructing a JSON data.
function test1(p) {
Log("Calls a custom function with parameters:", p);
return p;
}
function main() {
while (true) {
var table = {
type: 'table',
title: 'position operation',
cols: ['Column1', 'Column2', 'Action'],
rows: [
['a', '1', {
'type': 'button', // To display a button, you must set the type to button.
'cmd': "CoverAll", // String, sent data, accepted by the GetCommand() function.
'name': 'close position' // The name displayed on the button.
}],
['b', '1', {
'type': 'button',
'cmd': 10, // numerical value
'name': 'Send value'
}],
['c', '1', {
'type': 'button',
'cmd': _D(), // The function is called for the duration of the strategy run
'name': 'call the function'
}],
['d', '1', {
'type': 'button',
'cmd': 'JScode:test1("ceshi")', // String, the JS code to execute.
'name': 'Send JS Code'
}]
]
};
LogStatus('`' + JSON.stringify(table) + '`')
var str_cmd = GetCommand();
if (str_cmd) {
Log("Received Interaction Data str_cmd:", "type:", typeof(str_cmd), "value:", str_cmd);
}
if (str_cmd && str_cmd.split(':', 2)[0] == "JScode") { // Determine if there is a message
var js = str_cmd.split(':', 2)[1]; // Split the returned message string, limit it to two, and assign the element with index 1 to a variable named js.
Log("Execute debugging code:", js); // Output executed code
try { // Abnormal detection
eval(js); // Executes the eval function, which executes the parameters (code) passed in.
} catch (e) { // throw an exception
Log("Exception", e); // Output error messages
}
}
Sleep(500);
}
}
Let’s run it. The strategy runs as shown:
We can trigger the interaction by clicking on the buttons in the table on the status bar. We will click on the “Close Position” and “Send Value” buttons in turn. When we click on the “Close Position” button, the message will be sent as normal:
> But it doesn't work when you click on "Send value" because[ ```'cmd': 10, // value``` ]Here is 10. Numeric types cannot be sent.
https://www.fmz.com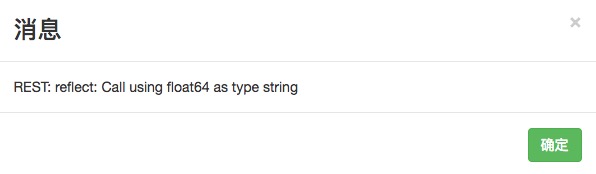
# It has been optimized to be compatible with numeric values, and returns a string of values.
Next we click on the “Call Function” button, to test the function being called is the _D() function, and the _D() function will keep returning the current time string, so if you write a function call here, it will keep calling it.
The received data is printed in the log:
Finally, let’s click on the “Send JS Code” button and we can execute the custom function that we used to test in our code.
function test1(p) {
Log("Calls a custom function with parameters:", p);
return p;
}
Click the button:
You can see that the Log("Calling custom function with parameters: ", p); statement in function test1 was executed.
Inserting ‘class’: ‘btn btn-xs btn-danger’, style in the code changes the appearance of the button.
Get started and practice right away!