发明者量化开放了平台的扩展API接口,支持程序化调用发明者量化交易平台的各项功能。
发明者量化交易平台支持扩展API接口的权限管理,可以设置API KEY
的权限。在平台账号设置页面的「API接口」选项,点击「创建新的ApiKey」按钮创建扩展API KEY
。
可以在创建API KEY
时编辑「API权限」输入框,输入*
符号开启所有扩展API接口权限。如果要指定具体接口权限,需要输入对应的扩展API函数名。使用逗号间隔,例如:GetRobotDetail,DeleteRobot
。即给予了这个API KEY
调用获取实盘详细信息接口、删除实盘接口的权限。
在API KEY
管理页面也可以对已经创建的API KEY
进行修改、禁用、删除操作。
扩展API接口返回的结构举例如下:
{
"code":0,
"data":{
// ...
}
}
| 描述 | 代码 |
| - | - |
| 执行成功 | 0 |
| 错误的API KEY| 1 |
| 错误的签名 | 2 |
| Nonce错误 | 3 |
| 方法不正确 | 4 |
| 参数不正确 | 5 |
| 内部未知错误 | 6 |
### 实盘状态码
```GetRobotList```接口、```GetRobotDetail```接口、```GetRobotLogs```接口返回的数据中```status```字段为:实盘状态码。
- 正常启动
| 状态 | 代码 |
| - | - |
| 空闲中 | 0 |
| 运行中 | 1 |
| 停止中 | 2 |
| 已退出 | 3 |
| 被停止 | 4 |
| 策略有错误 | 5 |
- 异常
| 状态 | 代码 |
| - | - |
| 策略已过期,请联系作者重新购买 | -1 |
| 没有找到托管者 | -2 |
| 策略编译错误 | -3 |
| 实盘已经是运行状态 | -4 |
| 余额不足 | -5 |
| 策略并发数超限 | -6 |
### 验证方式
调用扩展API接口时有两种验证方式,支持```token```验证和直接验证。
### token验证
使用```md5```加密方式验证,```Python```、```Golang```语言调用例子:
```python
#!/usr/bin/python
# -*- coding: utf-8 -*-
import time
import json
import ssl
ssl._create_default_https_context = ssl._create_unverified_context
try:
import md5
import urllib2
from urllib import urlencode
except:
import hashlib as md5
import urllib.request as urllib2
from urllib.parse import urlencode
accessKey = '' # your API KEY
secretKey = ''
def api(method, *args):
d = {
'version': '1.0',
'access_key': accessKey,
'method': method,
'args': json.dumps(list(args)),
'nonce': int(time.time() * 1000),
}
d['sign'] = md5.md5(('%s|%s|%s|%d|%s' % (d['version'], d['method'], d['args'], d['nonce'], secretKey)).encode('utf-8')).hexdigest()
# 注意: urllib2.urlopen 函数,超时问题,可以设置超时时间,urllib2.urlopen('https://www.fmz.com/api/v1', urlencode(d).encode('utf-8'), timeout=10) 设置超时 10秒
return json.loads(urllib2.urlopen('https://www.fmz.com/api/v1', urlencode(d).encode('utf-8')).read().decode('utf-8'))
# 返回托管者列表
print(api('GetNodeList'))
# 返回交易所列表
print(api('GetPlatformList'))
# GetRobotList(offset, length, robotStatus, label),传-1代表获取全部
print(api('GetRobotList', 0, 5, -1, 'member2'))
# CommandRobot(robotId, cmd)向实盘发送命令
print(api('CommandRobot', 123, 'ok'))
# StopRobot(robotId)返回实盘状态代码
print(api('StopRobot', 123))
# RestartRobot(robotId)返回实盘状态代码
print(api('RestartRobot', 123))
# GetRobotDetail(robotId)返回实盘详细信息
print(api('GetRobotDetail', 123))
package main
import (
"fmt"
"time"
"encoding/json"
"crypto/md5"
"encoding/hex"
"net/http"
"io/ioutil"
"strconv"
"net/url"
)
// 填写自己的FMZ平台api key
var apiKey string = ""
// 填写自己的FMZ平台secret key
var secretKey string = ""
var baseApi string = "https://www.fmz.com/api/v1"
func api(method string, args ... interface{}) (ret interface{}) {
// 处理args
jsonStr, err := json.Marshal(args)
if err != nil {
panic(err)
}
params := map[string]string{
"version" : "1.0",
"access_key" : apiKey,
"method" : method,
"args" : string(jsonStr),
"nonce" : strconv.FormatInt(time.Now().UnixNano() / 1e6, 10),
}
data := fmt.Sprintf("%s|%s|%s|%v|%s", params["version"], params["method"], params["args"], params["nonce"], secretKey)
h := md5.New()
h.Write([]byte(data))
sign := h.Sum(nil)
params["sign"] = hex.EncodeToString(sign)
// http request
client := &http.Client{}
// request
urlValue := url.Values{}
for k, v := range params {
urlValue.Add(k, v)
}
urlStr := urlValue.Encode()
request, err := http.NewRequest("GET", baseApi + "?" + urlStr, nil)
if err != nil {
panic(err)
}
resp, err := client.Do(request)
if err != nil {
panic(err)
}
defer resp.Body.Close()
b, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
ret = string(b)
return
}
func main() {
settings := map[string]interface{}{
"name": "hedge test",
"strategy": 104150,
// K线周期参数,60即为60秒
"period": 60,
"node" : 73938,
"appid": "member2",
"exchanges": []interface{}{
map[string]interface{}{
"eid": "Exchange",
"label" : "test_bjex",
"pair": "BTC_USDT",
"meta" : map[string]interface{}{
// 填写access key
"AccessKey": "",
// 填写secret key
"SecretKey": "",
"Front" : "http://127.0.0.1:6666/exchange",
},
},
},
}
method := "RestartRobot"
fmt.Println("调用接口:", method)
ret := api(method, 124577, settings)
fmt.Println("main ret:", ret)
}
支持不使用token
验证(直接传secret_key
验证),可以生成一个用来直接访问的URL。例如直接给实盘发交互指令的URL,可以用于Trading View
或其它场景的WebHook
回调。对于扩展API接口CommandRobot()
函数,不进行nonce
校验,不限制该接口的访问频率、访问次数。
例如:创建的扩展API KEY
中的AccessKey
为:xxx
,SecretKey
为:yyy
。访问以下链接即可向实盘Id为186515
的实盘发送交互指令消息,消息内容为字符串:"ok12345"
。
https://www.fmz.com/api/v1?access_key=xxx&secret_key=yyy&method=CommandRobot&args=[186515,"ok12345"]
支持直接验证方式下,获取请求中的Body
数据,仅支持CommandRobot
接口。例如在Trading View
的WebHook URL
中设置:
https://www.fmz.com/api/v1?access_key=xxx&secret_key=yyy&method=CommandRobot&args=[186515,+""]
注意需要按照此格式设置:args=[186515,+""]
,186515
是发明者量化交易平台的实盘Id。Trading View
消息框中设置(要发送的请求中的Body数据):
https://www.fmz.com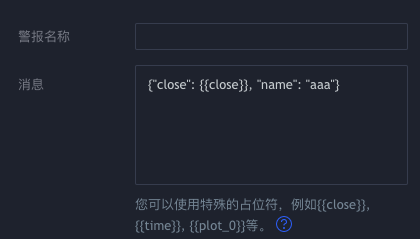
{"close": {{close}}, "name": "aaa"}
- 文本格式:
https://www.fmz.com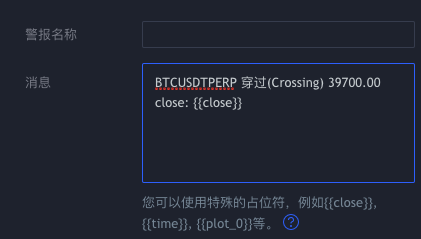
```plaintext
BTCUSDTPERP 穿过(Crossing) 39700.00 close: {{close}}
```Python```、```Golang```语言调用例子:
```python
#!/usr/bin/python
# -*- coding: utf-8 -*-
import json
import ssl
ssl._create_default_https_context = ssl._create_unverified_context
try:
import urllib2
except:
import urllib.request as urllib2
accessKey = 'your accessKey'
secretKey = 'your secretKey'
def api(method, *args):
return json.loads(urllib2.urlopen(('https://www.fmz.com/api/v1?access_key=%s&secret_key=%s&method=%s&args=%s' % (accessKey, secretKey, method, json.dumps(list(args)))).replace(' ', '')).read().decode('utf-8'))
# 如果API KEY没有该接口权限,调用print(api('RestartRobot', 186515)) 会失败,返回数据:{'code': 4, 'data': None}
# print(api('RestartRobot', 186515))
# 打印Id为:186515的实盘详细信息
print(api('GetRobotDetail', 186515))
package main
import (
"fmt"
"encoding/json"
"net/http"
"io/ioutil"
"net/url"
)
// 填写自己的FMZ平台api key
var apiKey string = "your access_key"
// 填写自己的FMZ平台secret key
var secretKey string = "your secret_key"
var baseApi string = "https://www.fmz.com/api/v1"
func api(method string, args ... interface{}) (ret interface{}) {
jsonStr, err := json.Marshal(args)
if err != nil {
panic(err)
}
params := map[string]string{
"access_key" : apiKey,
"secret_key" : secretKey,
"method" : method,
"args" : string(jsonStr),
}
// http request
client := &http.Client{}
// request
urlValue := url.Values{}
for k, v := range params {
urlValue.Add(k, v)
}
urlStr := urlValue.Encode()
request, err := http.NewRequest("GET", baseApi + "?" + urlStr, nil)
if err != nil {
panic(err)
}
resp, err := client.Do(request)
if err != nil {
panic(err)
}
defer resp.Body.Close()
b, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
ret = string(b)
return
}
func main() {
method := "GetRobotDetail"
fmt.Println("调用接口:", method)
ret := api(method, 186515)
fmt.Println("main ret:", ret)
}
使用发明者量化交易平台扩展API实现TradingView报警信号交易 使用发明者量化交易平台扩展API实现TradingView报警信号交易,B站视频链接
https://www.fmz.com/api/v1
后直接附加请求的查询参数(以?
分隔),以下是使用Python
表达的请求参数: {
"version" : "1.0",
"access_key": "xxx",
"method" : "GetNodeList",
"args" : [],
"nonce" : 1516292399361,
"sign" : "085b63456c93hfb243a757366600f9c2"
}
| 字段 | 说明 | | - | - | | version | 版本号。 | | access_key | AccessKey,在账户管理页面申请。 | | method | 具体调用的方法。 | | args | 具体调用的method方法的参数列表。 | | nonce | 时间戳,单位毫秒,允许和标准时间时间戳前后误差1小时,nonce必须比上一次访问时的nonce数值大。 | | sign | 签名。 |
各个参数以字符&
分隔,参数名和参数值用符号=
连接,完整的请求URL(method=GetNodeList
为例):
https://www.fmz.com/api/v1?access_key=xxx&nonce=1516292399361&args=%5B%5D&sign=085b63456c93hfb243a757366600f9c2&version=1.0&method=GetNodeList
注意请求参数中没有secret_key
这个参数。
- 签名方式
请求参数中sign
参数加密方式如下,按照格式:
version + "|" + method + "|" + args + "|" + nonce + "|" + secretKey
拼接字符串后,使用MD5
加密算法加密字符串,并转换为十六进制数据字符串,该值作为参数sign
的值。签名部分参考Python
代码扩展API接口「验证方式」:
# 参数
d = {
'version': '1.0',
'access_key': accessKey,
'method': method,
'args': json.dumps(list(args)),
'nonce': int(time.time() * 1000),
}
# 计算sign签名
d['sign'] = md5.md5(('%s|%s|%s|%d|%s' % (d['version'], d['method'], d['args'], d['nonce'], secretKey)).encode('utf-8')).hexdigest()
{
"code":0,
"data":{
"result":null,
"error":"Params length incorrect"
}
}
```JSON
{
"code": 0,
"data": {
"result": {
"all": 1,
"nodes": [{
"build": "3.7",
"city": "...",
"created": "2024-11-08 09:21:08",
"date": "2024-11-08 16:37:16",
"forward": "...",
"guid": "...",
"host": "node.fmz.com:9902",
"id": 123,
"ip": "...",
"is_owner": true,
"loaded": 0,
"name": "MacBook-Pro-2.local",
"online": true,
"os": "darwin/amd64",
"peer": "...",
"public": 0,
"region": "...",
"tunnel": false,
"version": "...",
"wd": 0
}]
},
"error": null
}
}
返回值字段说明(字面意思较明显的不再赘述):
- all: 当前账户关联的托管者数量。
- nodes: 记录托管者节点详细信息。
- build: 版本号。
- city: 所在城市。
- is_owner: true表示是私有托管者,false表示是公共托管者。
- loaded: 负载,搭载策略实例的个数。
- public: 0表示私有托管者,1表示公共托管者。
- region: 地理位置。
- version: 托管者详细版本信息。
- wd: 是否开启离线报警,0表示未开启。
一键部署的托管者包含一些额外信息,字段以ecs_
、unit_
前缀开头,记录了一键部署托管者服务器的相关信息(运营商名称、配置、状态等),计费周期、价格等信息,不再赘述。
无参数
```JSON
{
"code": 0,
"data": {
"result": {
"items": [{
"id": 3417,
"name": "测试"
}, {
"id": 3608,
"name": "实盘演示"
}]
},
"error": null
}
}
无参数
### GetPlatformList
```GetPlatformList```方法用于获取请求中的```API KEY```对应的发明者量化交易平台账号下的已添加的交易所列表。
```JSON
{
"code": 0,
"data": {
"result": {
"all": 2,
"platforms": [{
"category": "加密货币||Crypto",
"date": "2023-12-07 13:44:52",
"eid": "Binance",
"id": 123,
"label": "币安",
"logo": "...",
"name": "币安现货|Binance",
"stocks": ["BTC_USDT", "LTC_USDT", "ETH_USDT", "ETC_USDT", "BTC_TUSD", "ETH_TUSD", "BNB_TUSD"],
"website": "..."
}, {
"category": "通用协议|Custom Protocol",
"date": "2020-11-09 11:23:48",
"eid": "Exchange",
"id": 123,
"label": "XX交易所REST协议",
"logo": "...",
"name": "通用协议|Custom Protocol",
"stocks": ["BTC_USDT", "ETH_USDT"],
"website": ""
}]
},
"error": null
}
}
eid
。无参数
```JSON
{
"code": 0,
"data": {
"result": {
"all": 1,
"concurrent": 0,
"robots": [{
"charge_time": 1731654846,
"date": "2024-11-12 14:05:29",
"end_time": "2024-11-15 14:56:32",
"fixed_id": 4509153,
"id": 591026,
"is_sandbox": 0,
"name": "测试",
"node_guid": "45891bcf3d57f99b08a43dff76ee1ea1",
"node_id": 4519153,
"node_public": 0,
"profit": 0,
"public": 0,
"refresh": 1731651257000,
"start_time": "2024-11-15 14:56:30",
"status": 3,
"strategy_id": 411670,
"strategy_isowner": true,
"strategy_language": 0,
"strategy_name": "测试",
"strategy_public": 0,
"uid": "105ed6e511cc977921610fdbb7e2a1d6",
"wd": 0
}]
},
"error": null
}
}
group_id
字段。分页查询偏移设置。
offset
false
number
分页查询长度设置。
length
false
number
指定所要查询的实盘状态,参考扩展API接口「实盘状态码」,传-1
代表获取全部实盘。
robotStatus
false
number
指定所要查询的实盘自定义标签,可以筛选出这个标签的所有实盘。
label
false
string
查询关键字。
keyWord
false
string
以Python
语言的扩展API接口「验证方式」为例:
```print(api('GetRobotList', 'member2'))```:把自定义标签为member2的实盘信息全部打印出来。
```print(api('GetRobotList', 0, 5, -1, 'member2'))```:分页从0到5列出来最多5个标签为member2的实盘。
### CommandRobot
```CommandRobot```方法用于向请求中的```API KEY```对应的发明者量化交易平台账号下的实盘发送交互命令,接收交互命令的实盘Id为```robotId```参数指定的实盘Id,交互命令由策略中调用的```GetCommand()```函数捕获返回。
```JSON
{
"code":0,
"data":{
"result":true,
"error":null
}
}
robotId
true
number
```cmd```参数是发送给实盘的交互指令,在实盘策略中的```GetCommand()```函数会捕获到该交互命令,触发策略的交互逻辑。在策略代码中具体实现交互逻辑,可以参看[发明者量化交易平台API手册](https://www.fmz.com/syntax-guide#fun_getcommand)中的```GetCommand()```函数。
cmd
true
string
实盘策略,假设这个策略实盘处于运行中,实盘Id为123:
```js
function main() {
while (true) {
var cmd = GetCommand()
if (cmd) {
Log(cmd)
}
Sleep(2000)
}
}
如果使用本章节的Python测试脚本,访问发明者量化交易平台的扩展API:api("CommandRobot", 123, "test command")
。Id为123的实盘会收到交互指令:test command
,然后通过Log函数输出打印出来。
```JSON
{
"code":0,
"data":{
"result":2,
"error":null
}
}
robotId
true
number
### RestartRobot
```RestartRobot```方法用于重启请求中的```API KEY```对应的发明者量化交易平台账号下的实盘,重启的实盘Id为```robotId```参数指定的实盘Id。
```JSON
{
"code":0,
"data":{
"result":1,
"error":null
}
}
robotId
true
number
实盘配置参数,```settings```参数格式如下:
```JSON
{
"appid":"test",
"args":[],
"exchanges":[
{"pair":"SOL_USDT","pid":123},
{"pair":"ETH_USDT","pid":456}
],
"name":"测试",
"node":123,
"period":60,
"strategy":123
}
Interval
,重启策略时希望Interval
设置为500,则args
中包含:["Interval", 500]
,即:"args": [["Interval", 500]]
。pid
配置:{"pair":"SOL_USDT","pid":123}
;pid
可以通过GetPlatformList
接口查询,返回的数据中id
字段即为交易所pid
。eid
配置:{"eid":"Huobi","label":"test Huobi","meta":{"AccessKey":"123","SecretKey":"123"},"pair":"BCH_BTC"}
;传入的API KEY
之类的敏感信息,发明者量化交易平台是不储存的,这些数据直接转发给托管者程序。如果使用此类配置,每次创建或者重启实盘时必须配置该信息。{"eid":"Exchange","label":"test exchange","pair":"BTC_USDT","meta":{"AccessKey":"123","SecretKey":"123","Front":"http://127.0.0.1:6666/test"}}
。label
属性是给当前通用协议接入的交易所对象设置一个标签,在策略中可以使用exchange.GetLabel()
函数获取。GetStrategyList
方法获取到。settings false JSON对象
如果是使用扩展API接口创建出的实盘,重启必须使用扩展API接口RestartRobot
进行重启,并且必须传入settings
参数。在平台页面上创建的实盘,可以通过扩展API接口重启或者点击实盘页面上的按钮重启,可以传settings
参数或者不传settings
参数,只传robotId
这个参数,如果只传robotId
参数,则按照当前实盘的设置启动实盘运行。
```JSON
{
"code": 0,
"data": {
"result": {
"robot": {
"charge_time": 1732246539,
"charged": 5850000,
"consumed": 5375000000,
"date": "2018-12-28 14:34:51",
"favorite": {
"added": false,
"type": "R"
},
"fixed_id": 123,
"hits": 1,
"id": 123,
"is_deleted": 0,
"is_manager": true,
"is_sandbox": 0,
"name": "测试",
"node_id": 123,
"pexchanges": {
"123": "Futures_OKCoin"
},
"phash": {
"123": "ca1aca74b9cf7d8624f2af2dac01e36d"
},
"plabels": {
"123": "OKEX期货 V5"
},
"priority": 0,
"profit": 0,
"public": 0,
"refresh": 1732244453000,
"robot_args": "[]",
"start_time": "2024-11-22 11:00:48",
"status": 1,
"strategy_args": "[]",
"strategy_exchange_pairs": "[60,[123],[\"ETH_USDT\"]]",
"strategy_id": 123,
"strategy_last_modified": "2024-11-21 16:49:25",
"strategy_name": "测试",
"strategy_public": "0",
"uid": "105ed6e51bcc17792a610fdbb7e2a1d6",
"username": "abc",
"wd": 0
}
},
"error": null
}
}
robotId
true
number
```strategy_exchange_pairs```属性说明,用以下数据为例:
```plaintext
"[60,[44314,42960,15445,14703],[\"BTC_USDT\",\"BTC_USDT\",\"ETH_USDT\",\"ETH_USDT\"]]"
其中第一个数据60
,代表实盘设置的默认K线周期为1分钟,即60秒。
```[\"BTC_USDT\",\"BTC_USDT\",\"ETH_USDT\",\"ETH_USDT\"]```为实盘配置的交易所对象设置的交易对(按添加顺序与pid一一对应)。
### GetAccount
```GetAccount```方法用于获取请求中的```API KEY```对应的发明者量化交易平台账号的账户信息。
```JSON
{
"code":0,
"data":{
"result":{
"balance":22944702436,
"concurrent":0,
"consumed":211092719653,
"currency":"USD",
"email":"123@qq.com",
"openai":false,
"settings":null,
"sns":{"wechat":true},
"uid":"105ea6e51bcc177926a10fdbb7e2a1d6",
"username":"abc"
},
"error":null
}
}
```isSummary```参数为```false```时,返回的数据:
```JSON
{
"code": 0,
"data": {
"result": {
"exchanges": [{
"category": "加密货币||Crypto",
"eid": "Futures_Binance",
"id": 74,
"logo": "/upload/asset/d8d84b23e573e9326b99.svg",
"meta": "[{\"desc\": \"Access Key\", \"qr\":\"apiKey\",\"required\": true, \"type\": \"string\", \"name\": \"AccessKey\", \"label\": \"Access Key\"}, {\"encrypt\": true, \"qr\":\"secretKey\",\"name\": \"SecretKey\", \"required\": true, \"label\": \"Secret Key\", \"type\": \"password\", \"desc\": \"Secret Key\"}]",
"name": "币安期货|Futures_Binance",
"priority": 200,
"stocks": "BTC_USDT,ETH_USDT,ETH_USD",
"website": "https://accounts.binance.com/zh-TC/register?ref=45110270"
}]
},
"error": null
}
}
```JSON
{
"code": 0,
"data": {
"result": {
"exchanges": [{
"category": "加密货币||Crypto",
"eid": "Futures_Binance",
"id": 74,
"logo": "/upload/asset/d8d84b23e573e9326b99.svg",
"name": "币安期货|Futures_Binance",
"priority": 200,
"website": "https://accounts.binance.com/zh-TC/register?ref=45110270"
}]
},
"error": null
}
}
isSummary
true
bool
### DeleteNode
```DeleteNode```方法用于删除请求中的```API KEY```对应的发明者量化交易平台账号的托管者节点,删除的托管者节点Id为```nid```参数指定的托管者Id。
```JSON
{
"code":0,
"data":{
"result":true,
"error":null
}
}
nid
true
number
### DeleteRobot
```DeleteRobot```方法用于删除请求中的```API KEY```对应的发明者量化交易平台账号下的实盘,删除的实盘Id为```robotId```参数指定的实盘Id。
```JSON
{
"code":0,
"data":{
"result":0,
"error":null
}
}
robotId
true
number
```deleteLogs```参数用于设置是否要删除实盘日志,如果传入真值(例如:```true```)即删除实盘日志。
deleteLogs
true
bool
### GetStrategyList
```GetStrategyList```方法用于获取平台策略信息。
```JSON
{
"code": 0,
"data": {
"result": {
"all": 123,
"strategies": [{
"category": 9,
"date": "2024-11-10 20:40:04",
"description": "",
"forked": 0,
"hits": 0,
"id": 123,
"is_buy": false,
"is_owner": false,
"language": 2,
"last_modified": "2024-11-11 17:23:52",
"name": "HedgeGridStrategy",
"profile": {
"avatar": "...",
"nickname": "abc",
"uid": "4ed225440db1eda23fe05ed10184113e"
},
"public": 0,
"tags": "",
"uid": "4ed225440db1eda23fe05ed10184113e",
"username": "abc"
}]
},
"error": null
}
}
offset
true
number
```length```参数用于设置查询时的长度。
length
true
number
```strategyType```参数用于设置所要查询的策略类型。
- ```strategyType```参数设置```0```: 所有策略。
- ```strategyType```参数设置```1```: 已公开策略。
- ```strategyType```参数设置```2```: 待审核策略。
strategyType
true
number
```category```参数用于设置所要查询的策略种类。
- ```category```参数设置```-1```: 所有策略。
- ```category```参数设置```0```: 通用策略。
category
true
number
```needArgs```参数用于设置所要查询的策略是否有参数。
- ```needArgs```参数设置```0```: 所有策略。
needArgs
true
number
```language```参数用于设置所要查询的策略的编程语言。
- ```language```参数设置```0```: JavaScript语言。
- ```language```参数设置```1```: Python语言。
- ```language```参数设置```2```: C++语言。
- ```language```参数设置```3```: 可视化策略。
- ```language```参数设置```4```: My语言。
- ```language```参数设置```5```: PINE语言。
language
true
number
```kw```参数用于设置所要查询的策略的关键字。
- 设置空字符串即不使用关键字筛选。
kw
true
string
### NewRobot
```NewRobot```方法用于创建一个请求中的```API KEY```对应的发明者量化交易平台账号下的实盘。
```JSON
{
"code":0,
"data":{
"result":591988,
"error":null
}
}
实盘配置参数,settings
参数格式如下:
{
"appid":"test",
"args":[],
"exchanges":[
{"pair":"SOL_USDT","pid":123}
],
"group":123,
"name":"test",
"node":123,
"period":60,
"strategy":123
}
RestartRobot
接口。settings true JSON对象
如果创建使用通用协议交易所对象的实盘,在配置```settings```参数时,对于```exchanges```属性可以使用如下设置:
```JSON
{
"eid": "Exchange",
"label": "test",
"pair": "ETH_BTC",
"meta": {
"AccessKey": "123",
"SecretKey": "123",
"Front": "http://127.0.0.1:6666/test"
}
}
### PluginRun
```PluginRun```方法用于调用发明者量化交易平台的**调试工具**功能;仅支持JavaScript语言。
```JSON
{
"code": 0,
"data": {
"result": "{\"logs\":[{\"PlatformId\":\"\",\"OrderId\":\"0\",\"LogType\":5,\"Price\":0,\"Amount\":0,\"Extra\":\"Hello FMZ\",\"Currency\":\"\",\"Instrument\":\"\",\"Direction\":\"\",\"Time\":1732267473108}],\"result\":\"\"}",
"error": null
}
}
调试工具中的设置参数,settings
配置中包括了测试代码,在source
属性中。settings
参数格式如下:
{
"exchanges":[{"pair":"SOL_USDT","pid":123}],
"node":123,
"period":60,
"source":"function main() {Log(\"Hello FMZ\")}"
}
RestartRobot
接口。settings true JSON对象
{"eid": "OKEX", "pair": "ETH_BTC", "meta" :{"AccessKey": "123", "SecretKey": "123"}}
{"eid": "Huobi", "pair": "BCH_BTC", "meta" :{"AccessKey": "123", "SecretKey": "123"}}
对于settings
中的exchanges
属性来说,在调用PluginRun
方法时只用设置一个(在调试工具页面使用时也只支持一个交易所对象)。在settings
里设置2个交易所对象不会引起报错,但是在代码中如果访问第二个交易所对象就会报错。
```JSON
{
"code": 0,
"data": {
"result": {
"chart": "",
"chartTime": 0,
"logs": [{
"Total": 20,
"Max": 20,
"Min": 1,
"Arr": []
}, {
"Total": 0,
"Max": 0,
"Min": 0,
"Arr": []
}, {
"Total": 0,
"Max": 0,
"Min": 0,
"Arr": []
}],
"node_id": 123,
"online": true,
"refresh": 1732201544000,
"status": 4,
"summary": "...",
"updateTime": 1732201532636,
"wd": 0
},
"error": null
}
}
robotId
true
number
```logMinId```参数用于指定Log日志的最小Id。
logMinId
true
number
```logMaxId```参数用于指定Log日志的最大Id。
logMaxId
true
number
```logOffset```参数用于设置偏移,由```logMinId```和```logMaxId```确定范围后,根据```logOffset```偏移(跳过多少条记录),开始作为获取数据的起始位置。
logOffset
true
number
```logLimit```参数用于设置确定起始位置后,选取的数据记录条数。
logLimit
true
number
```profitMinId```参数用于设置收益日志的最小Id。
profitMinId
true
number
```profitMaxId```参数用于设置收益日志的最大Id。
profitMaxId
true
number
```profitOffset```参数用于设置偏移(跳过多少条记录),作为起始位置。
profitOffset
true
number
```profitLimit```参数用于设置确定起始位置后,选取的数据记录条数。
profitLimit
true
number
```chartMinId```参数用于设置图表数据记录的最小Id。
chartMinId
true
number
```chartMaxId```参数用于设置图表数据记录的最大Id。
chartMaxId
true
number
```chartOffset```参数用于设置偏移。
chartOffset
true
number
```chartLimit```参数用于设置获取的记录条数。
chartLimit
true
number
```chartUpdateBaseId```参数用于设置查询更新后的基础Id。
chartUpdateBaseId
true
number
```chartUpdateDate```参数用于设置数据记录更新时间戳,会筛选出比这个时间戳大的记录。
chartUpdateDate
true
number
```summaryLimit```参数用于设置查询的状态栏数据字节数。查询实盘的状态栏数据,该参数类型为整型。
设置0表示不需要查询状态栏信息,设置为非0表示需要查询的状态栏信息字节数(该接口不限制数据量,可以指定一个较大的summaryLimit参数来获取所有状态栏信息),状态栏数据储存在返回的数据的```summary```字段中。
summaryLimit
true
number
- 数据库中的策略日志表
返回数据中```logs```的属性值(数组结构)的第一个元素中(日志数据)```Arr```属性值描述如下:
```plaintext
"Arr": [
[3977, 3, "Futures_OKCoin", "", 0, 0, "Sell(688.9, 2): 20016", 1526954372591, "", ""],
[3976, 5, "", "", 0, 0, "OKCoin:this_week 仓位过多, 多: 2", 1526954372410, "", ""]
],
| id | logType | eid | orderId | price | amount | extra | date | contractType | direction | | - | - | - | - | - | - | - | - | - | - | | 3977 | 3 | “Futures_OKCoin” | “” | 0 | 0 | “Sell(688.9, 2): 20016” | 1526954372591 | “” | “” | | 3976 | 5 | “” | “” | 0 | 0 | “OKCoin:this_week 仓位过多, 多: 2” | 1526954372410 | “” | “” |
```logType```值具体代表的日志类型描述如下:
| logType: | 0 | 1 | 2 | 3 | 4 | 5 | 6 |
| - | - | - | - | - | - | - | - |
| logType意义: | BUY | SALE | RETRACT | ERROR | PROFIT | MESSAGE | RESTART |
| 中文意义 | 买单类型日志 | 卖单类型日志 | 撤销 | 错误 | 收益 | 日志 | 重启 |
- 数据库中的收益图表日志表
该图表日志表数据与策略日志表中的收益日志一致。
```plaintext
"Arr": [
[202, 2515.44, 1575896700315],
[201, 1415.44, 1575896341568]
]
以其中一条日志数据为例:
[202, 2515.44, 1575896700315]
- 数据库中的图表日志表
```plaintext
"Arr": [
[23637, 0, "{\"close\":648,\"high\":650.5,\"low\":647,\"open\":650,\"x\":1575960300000}"],
[23636, 5, "{\"x\":1575960300000,\"y\":3.0735}"]
]
以其中一条日志数据为例:
[23637, 0, "{\"close\":648,\"high\":650.5,\"low\":647,\"open\":650,\"x\":1575960300000}"],
23637
为日志Id,0
为图表数据系列索引,最后的数据"{\"close\":648,\"high\":650.5,\"low\":647,\"open\":650,\"x\":1575960300000}"
为日志数据,这条数据为图表上的K线数据。