The extended API of FMZ Quant Trading platform has recently been upgraded, and the upgrade supports the direct access mode, so that the TradingView alert signal can be easily sent to the bots on FMZ for automatic trading. If you don’t know what is an extended API is, now listen to me in detail.
Link of the Related Part in FMZ API Documentation
The main function of the extended API is to provide interfaces for various functions on FMZ Quant trading platform, for the programmatic operations, such as batch starting bots simultaneously, timing bot start and stop, reading bot information details, etc. We use the FMZ extended API to implement TradingView alert signal trading. This demand only needs to use the CommandRobot(RobotId, Cmd)
interface in the extended API. This interface can send interactive commands to the bot with the specified ID, and the bot can perform the corresponding operations (such as placing an order to buy or sell, etc.)
To use the extended API, you first need to create your own account API KEY
on FMZ:
### Direct Access Mode of Extended API
The direct access mode indicates directly writing ```API KEY``` in the Query of URL; for example, the URL accessing the extended API of FMZ Quant platform can be written as:
https://www.fmz.com/api/v1?access_key=xxx&secret_key=yyyy&method=CommandRobot&args=[186515,“ok12345”]
Among them, ```https://www.fmz.com/api/v1``` is the interface address; ```?``` is followed by ```Query```; the parameter ```access_key``` is, for example, represented by xxx (when using, fill in the access_key of your own FMZ account); the parameter ```secret_key``` is represented by yyyy (when using, fill in your own account secret_key); the parameter ```method``` is the specific name of the extended API interface to be accessed, and ```args``` is the parameter of the ```method``` interface to be called.
We use TradingView as a signal source to send trading commands to the FMZ bots. In fact, we only use the ```CommandRobot``` interface.
### TradingView
First of all, you need to have a TradingView Pro account. The Basic level cannot use the WebHood function in the alert. We enter the Chart of TradingView.
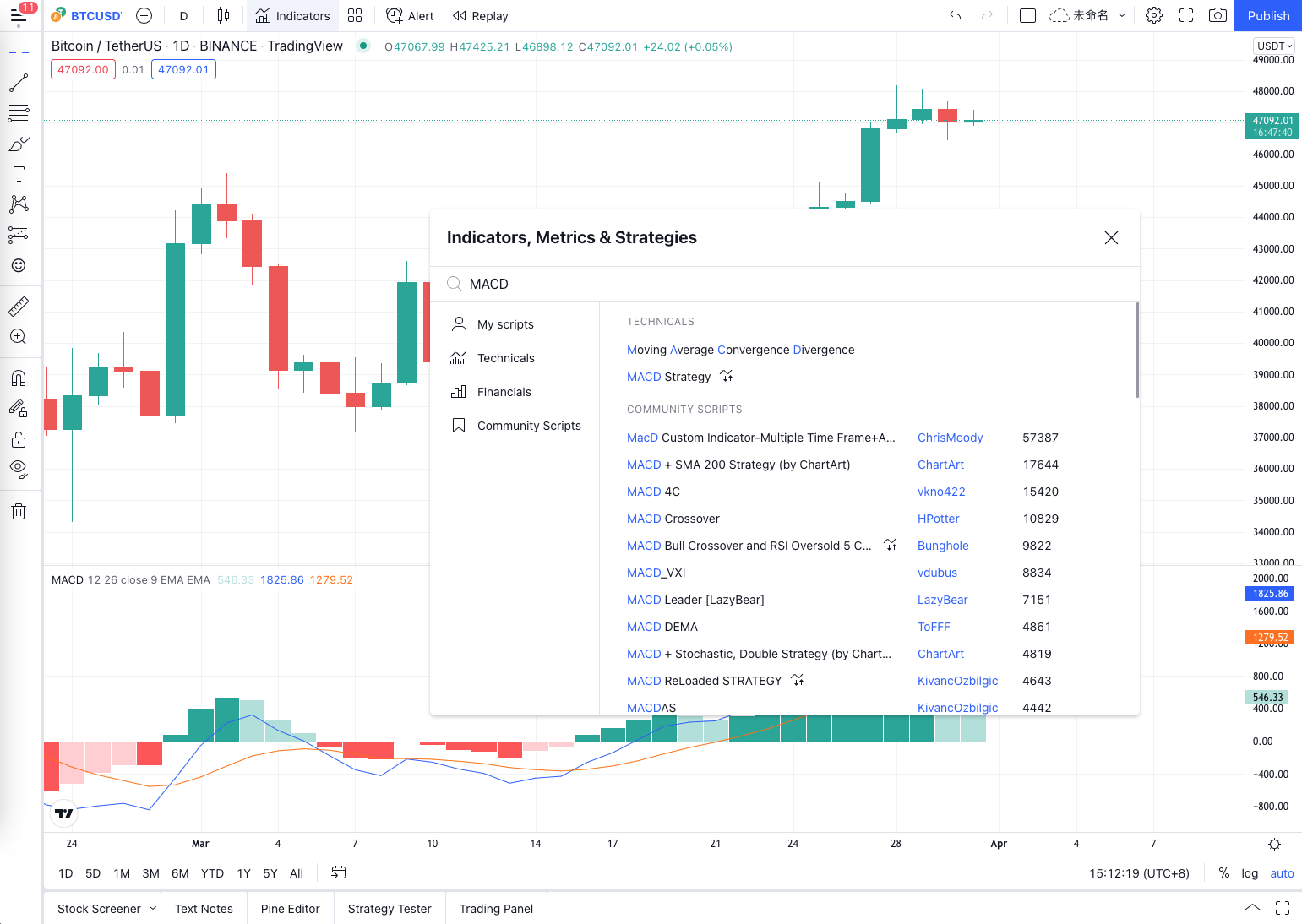
Add an indicator to the chart, and other script algorithms can also be used. Here, for the convenience of demonstration, we use the most commonly used ```MACD``` indicator, and then set the K-line period to 1 minute (in order to make the signal trigger faster and facilitate the demonstration).
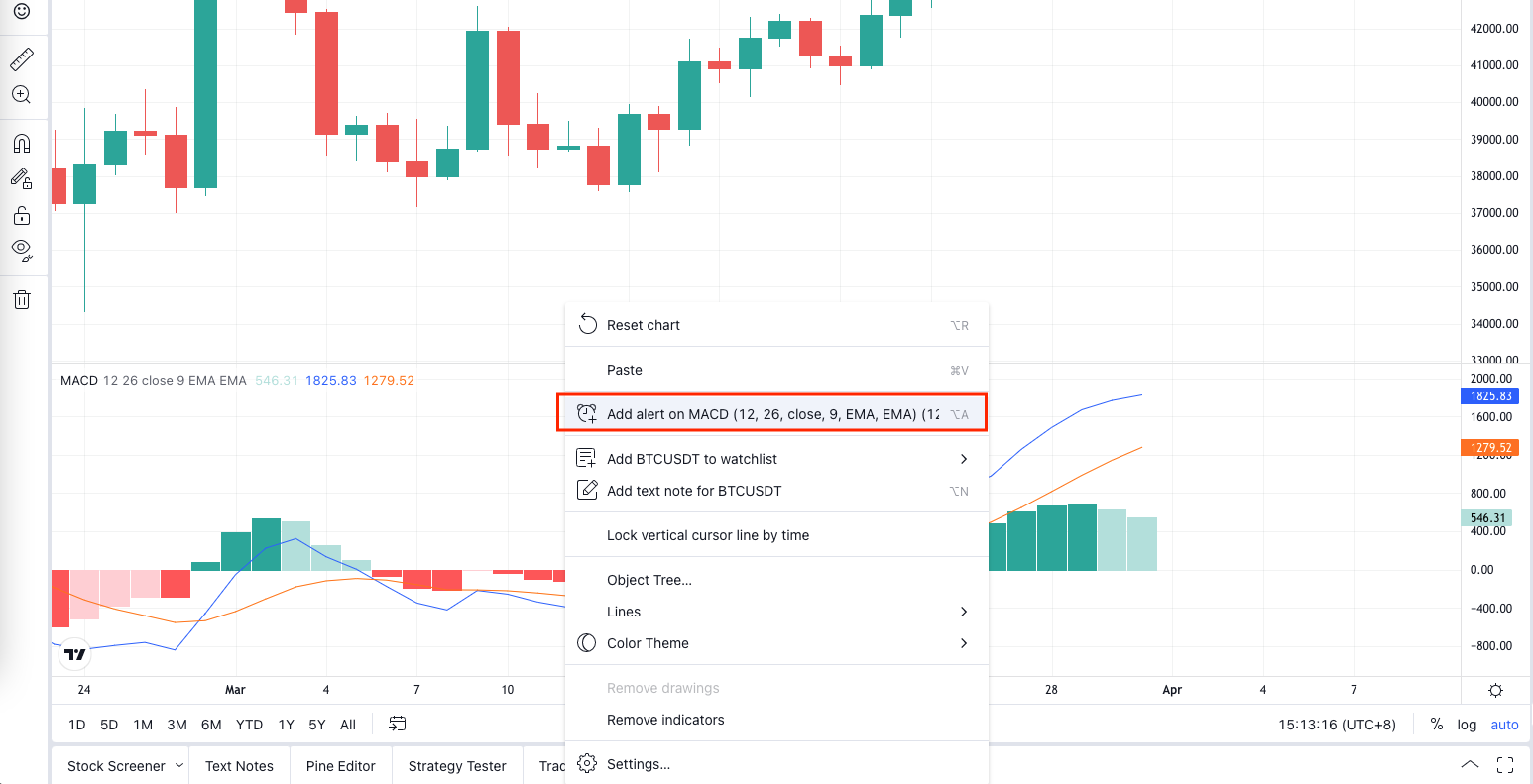
Right-click on the chart and select "Add Alert" from the pop-up menu.
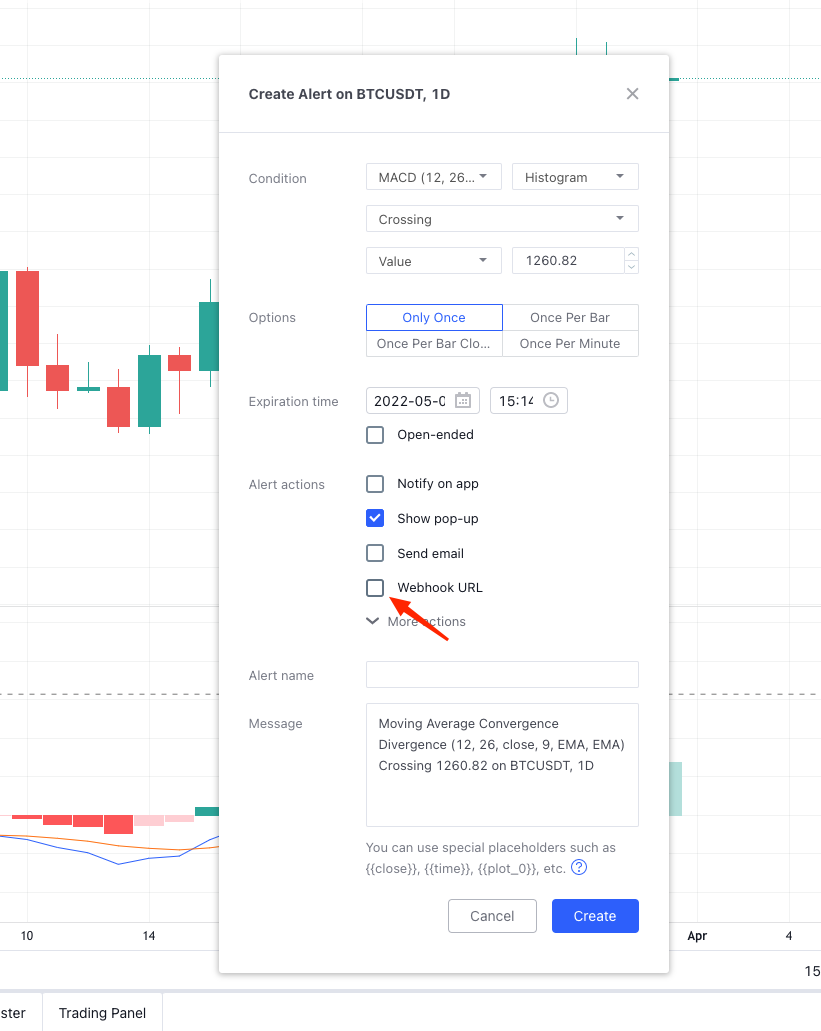
Set ```WebHook``` in the "Alert" pop-up window. At this point, you don't have to worry about setting it. Let's first run the bot that monitors the signals on FMZ Quant trading platform.
### Ordering Bot of Monitoring Signal
Strategy source code:
```js
// global variable
var BUY = "buy" // Note: the command used for spot
var SELL = "sell" // the command used for futures
var LONG = "long" // the command used for futures
var SHORT = "short" // the command used for futures
var COVER_LONG = "cover_long" // the command used for futures
var COVER_SHORT = "cover_short" // the command used for futures
function main() {
// Empty the logs; delete, if not needed
LogReset(1)
// Set the precision
exchange.SetPrecision(QuotePrecision, BasePrecision)
// Judge whether it is spot or futures
var eType = 0
var eName = exchange.GetName()
var patt = /Futures_/
if (patt.test(eName)) {
Log("The added platform is a futures platform:", eName, "#FF0000")
eType = 1
if (Ct == "") {
throw "Ct contract set to null"
} else {
Log(exchange.SetContractType(Ct), "Set contract:", Ct, "#FF0000")
}
} else {
Log("The added platform is a spot platform:", eName, "#32CD32")
}
var lastMsg = ""
var acc = _C(exchange.GetAccount)
while(true) {
var cmd = GetCommand()
if (cmd) {
// Detect the interactive command
lastMsg = "Command:" + cmd + "Time:" + _D()
var arr = cmd.split(":")
if (arr.length != 2) {
Log("Wrong cmd information:", cmd, "#FF0000")
continue
}
var action = arr[0]
var amount = parseFloat(arr[1])
if (eType == 0) {
if (action == BUY) {
var buyInfo = IsMarketOrder ? exchange.Buy(-1, amount) : $.Buy(amount)
Log("buyInfo:", buyInfo)
} else if (action == SELL) {
var sellInfo = IsMarketOrder ? exchange.Sell(-1, amount) : $.Sell(amount)
Log("sellInfo:", sellInfo)
} else {
Log("Spot trading platforms are not supported!", "#FF0000")
}
} else if (eType == 1) {
var tradeInfo = null
var ticker = _C(exchange.GetTicker)
if (action == LONG) {
exchange.SetDirection("buy")
tradeInfo = IsMarketOrder ? exchange.Buy(-1, amount) : exchange.Buy(ticker.Sell, amount)
} else if (action == SHORT) {
exchange.SetDirection("sell")
tradeInfo = IsMarketOrder ? exchange.Sell(-1, amount) : exchange.Sell(ticker.Buy, amount)
} else if (action == COVER_LONG) {
exchange.SetDirection("closebuy")
tradeInfo = IsMarketOrder ? exchange.Sell(-1, amount) : exchange.Sell(ticker.Buy, amount)
} else if (action == COVER_SHORT) {
exchange.SetDirection("closesell")
tradeInfo = IsMarketOrder ? exchange.Buy(-1, amount) : exchange.Buy(ticker.Sell, amount)
} else {
Log("Futures trading platforms are not supported!", "#FF0000")
}
if (tradeInfo) {
Log("tradeInfo:", tradeInfo)
}
} else {
throw "eType error, eType:" + eType
}
acc = _C(exchange.GetAccount)
}
var tbl = {
type : "table",
title : "Status information",
cols : ["Data"],
rows : []
}
// tbl.rows.push([JSON.stringify(acc)]) // Used during testing
LogStatus(_D(), eName, "The command received last time:", lastMsg, "\n", "`" + JSON.stringify(tbl) + "`")
Sleep(1000)
}
}
The code is very simple. It detects the return value of the GetCommand
function. When there is an interactive message sent to the strategy program, GetCommand
will return this message, and then the strategy program will make a corresponding trading operation based on the content of the message. The interaction button has been set up on the strategy, which can test the interactive function. For example, when the strategy is operated, the bot is configured with the simulated platform WexApp
of FMZ Quant trading platform.
Click the interaction button to test the bot capacity to receive an command to buy.
We can see the command string received by the bot is: buy:0.01
.
We only need to make the carried parameter be buy:0.01
during accessing the CommandRobot
interface of FMZ Quant extended API in the WebHook request URL, when the TradingView alert is triggered.
Back to TradingView, we fill the URL of the WebHook. Fill your own API KEY
in the access_key
and secret_key
parameters. For method
is fixed, we only need to access the extended API CommandRobot
; the args
parameter is in the form of [robot ID, command string]
, we can directly obtain the robot ID through the bot page, as shown in the figure:
This time when we trigger the signal, buy 0.02 coin, and the command string is: "buy:0.02"
. That completes the WebHook URL.
https://www.fmz.com/api/v1?access_key=e3809e173e23004821a9bfb6a468e308&secret_key=45a811e0009d91ad21154e79d4074bc6&method=CommandRobot&args=[443999,"buy:0.02"]
Set on TradingView:
Wait for the signal to be triggered. When the bot receives the signal, you can see the signal alert on the upper right of the page, and the trigger logs on the low right of the page.
The bot received the signal:
In this way, you can use the rich chart functions and indicator algorithms on TradingView to cooperate with the strategy bot of FMZ Quant to realize the automated trading you want. Compared with transplanting the strategies on TradingView into JavaScript and Python, the difficulty has reduced.
The strategy code of “Ordering Bot of Monitoring Signal” is only for study and research. It needs to be optimized and adjusted for the use of real bots. It also supports futures. We recommend to set it to market order mode. For details, please refer to the strategy code parameters. If you have any questions or suggestions, please leave a message.